Have you ever wondered "What is PHP?". You have certainly read or heard the term at least once. My experience shows that most of the time there is still little idea of what PHP is and how it works. This article should fill this knowledge gap and help you if the topic PHP is new to you and you still have little technical knowledge.
First and foremost, PHP is a scripting language. Developed in 1995 and originally called "Personal Home Page Tools", nowadays it is called "Hypertext Preprocessor", which is a more appropriate name because PHP generates server-side input for HTML - therefore, PHP makes HTML dynamic.
Alongside HTML, CSS and JavaScript, PHP is one of the internets' standard languages. Most people are able to avoid PHP when building their website, only because there are content management systems (CMS) like WordPress that offer the PHP part as a ready-to-use solution. But PHP teaches you the ability to modify your CMS (and thus WordPress in particular) in any way you want. This can be done in the form of the functions.php file or in the form of a plugin. If you've already learned a bit about WordPress, you'll have noticed that using plugins can enhance your website in many ways.
PHP versions
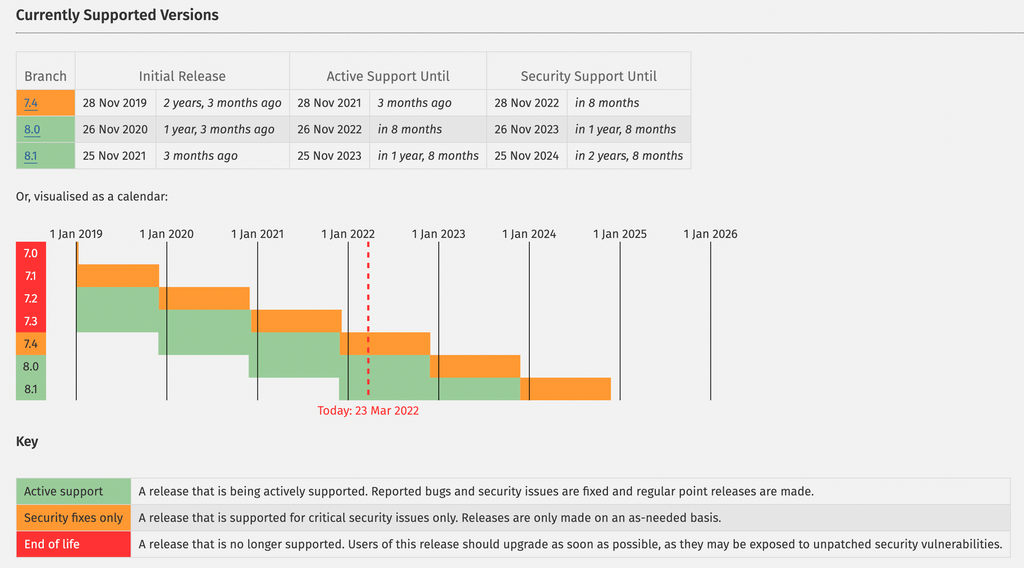
The latest version of PHP is 8.1. You can find which PHP versions are still actively supported and which are not on the official PHP website. Some host do not yet offer PHP 8 for customer websites. This is because this PHP version is not yet 100% compatible with WordPress or with all WordPress plugins.
When hosting WordPress with Raidboxes you can change the PHP version of your website with just one click - PHP 8 and PHP 8.1 are also available. Information on how to test a new PHP version properly can be found in our help center. If you have any further questions, feel free to contact us via Live Chat.
General concepts in PHP
As a link to the database, user inputs such as form contents are classically processed with PHP and the contents are used on the server side or entered directly into the database. If you have a contact form on your website, for example, the processing of a request will certainly be done via PHP.
PHP files can be integrated into other PHP files using the include command. This is useful to avoid redundant code and to separate certain functionalities. It also makes debugging easier, of course. This is also useful if the same element is to be used on several pages - the header, for example.
PHP - a server-side language
A peculiarity of PHP is that scripts are always executed on the server. If you have written a PHP script, you load the file onto your server, from where you can access it via your web browser by specifying the path there. This is similar to the pattern "Address of the server"/subfolder/filename.php. If you look at the address bar of your browser, many websites have the extension .html, but it also works with .php files.
The server has to be PHP-capable, of course. I.e. the PHP interpreter needs to be installed. But this is usually the standard for nowadays web servers.
Which also means, if you want to develop PHP, you must either have access to the file structure on your web server (via FTP) or you set up a local server with PHP for development. For this purpose, there is, among other things, the renownedXAMPPpackage, which was designed exactly for this purpose and contains everything you need for local development.
If you want to display a random number on your website, you create it in a PHP script, as HTML is not capable of performing such an operation on its own. In the following example, a random number between 1 and 5 is displayed on your website:

Dieses Beispiel demonstriert, dass hierbei Abschnitte geschrieben werden, die entweder aus PHP Code oder aus HTML Code bestehen und vom Server entsprechend interpretiert werden. PHP Code wird immer von den beiden Tags “<?php” und “?>” umschlossen. Alles, was sich außerhalb dieser Tags befindet, ist HTML Code und wird vom Server nur gesendet, aber nicht verarbeitet. Du verwendest die PHP Tags aber beim Schreiben des Codes genau so, wie du HTML Tags verwendest.
File extension
As soon as you want to execute even one line of PHP code, the file extension must be .php instead of .html. Only PHP code that is also in .php files will be executed! Therefore, you should pay attention to the file extension when using PHP.
The server executes the script when loading the file. This results in values for variables, in most cases. These are displayed in some way with HTML on the final website.
I am now doing the same with JavaScript:

JavaScript also generates dynamics on a website. The big difference is that the random digit in PHP can only be generated by the server and that the browser only receives the final result from the server. The browser is not affected by the underlying process. If you look at the sites' source code, you will see a digit in the HTML code that you could have entered manually. JavaScript, on the other hand, is executed on the client side (i.e. in the browser). Therefore, you can also view the JavaScript source code of the site in the browser.
PHP seems a bit chaotic to many, because it nests with HTML like that. Here there are so-called template engines that "separate" the PHP code from the HTML, such as Smarty, if you want to delve deeper into PHP. If you only want to use PHP in the WordPress context, such a thing is not necessary.
Data types in PHP
PHP is a weakly typed language. In simple terms, this means that the PHP interpreter finds out for itself what data type a variable is. In other, strongly typed, languages such as Java, the data types for each variable must normally be specified directly by the person at the computer in order for the programme to be executable at all. In PHP, a simple assignment is sufficient, as the following example demonstrates:

At this point, it is actually only important to understand that PHP normally sets the data type automatically. However, the data type of a variable can also be converted manually in the code using typecast. This follows certain rules and restrictions. This is beyond the scope of this article, unfortunately. - If you like to read more about it, I recommend this article on the official PHP website.
In short, the PHP interpreter recognises all content as corresponding data types. While this is very convenient and user-friendly, it is also, of course, a possible source of errors. My experience with PHP so far, however, is that errors in this regard occur rather rarely.
Objects and classes in PHP
A class can be used to describe certain objects. This is done via attributes, which are the properties of an object. With methods, certain behavior of the object can be represented - in other words, the question is "What is my object capable of?"
When creating objects, you always proceed in the same way - describe the object in general as a class using attributes and methods and then create an instance of this class at the required point in your code. Object orientation is often difficult to understand at first, so I'll explain briefly using the example of a ball:
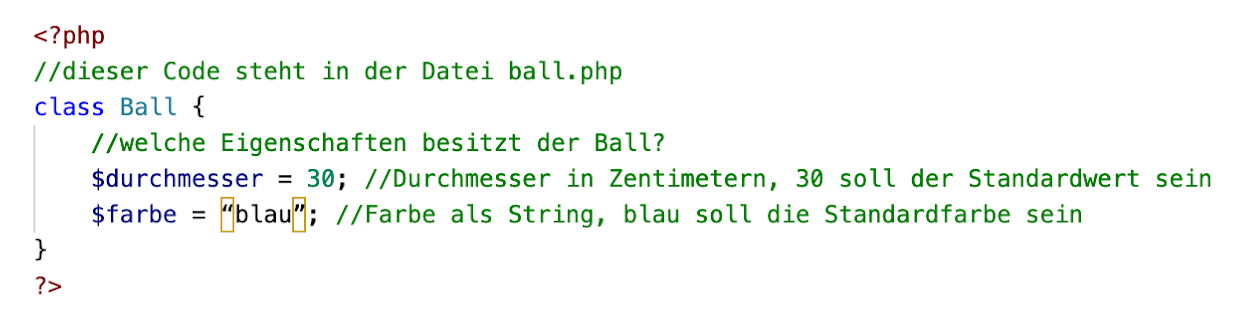
This part is saved as a fixed definition in the file ball.php. At this point, merely what a ball is (by default) and what it can do has been defined, a ball-object does not exist in your code yet. The ball is always created at the place (or in the file) where it is needed.

You now have created a red ball with a diameter of 20 cm. If you want to create the standard blue ball with 30 cm, it is sufficient to instantiate the object with:

To check the diameter of the ball, you could add this:

If you now call up this PHP file on your server using a browser, the diameter of the ball is output as a number.
What you do with this ball now is up to you. You want the ball to be displayed graphically and have two people kick it back and forth? Do you want the ball to be a dynamic style element on your website? Do you want to assign certain behavior to the ball via so-called methods, so that it can roll or be kicked, for example? There are many possibilities! Since this article is only intended to provide an introduction, I recommend further readinghere. Object orientation is a big topic of its own.
Arrays in PHP
If you don't know arrays already, they are like tables in which a certain content is assigned to a certain key. This content can be accessed via the key. It's kind of like writing a list of numbers and asking yourself "What is the third number in the list of numbers?" The answer to that would be the number in the third position. Or in other words - the content of the line with key 3.
Arrays also work a little differently in PHP than in other languages - they are implemented in the form of a so-called map. Again, in comparison to Java - an array needs a key in the form of a number in Java, for example myLittleArray[5]. Here, the "rows" are simply counted up starting with 0. In PHP, on the other hand, strings are used as keys to be able to assign terms to the content. For more details and examples, have a look here.
"*" indicates required fields
PHP in WordPress
WordPress too works using PHP. Most plugins are written in PHP themselves and access the backend and database of WordPress via PHP. You can read details about this in the official WordPress documentation. WordPress was designed in such a way that absolutely no PHP knowledge is required for the basic functionality. However, PHP is necessary for almost every individual customisation.
PHP troubleshooting
Errors can occur in every programming language, so some kind of debugging must be done in every language. PHP offers a number of convenient functions for this purpose.
error_log
This line allows debugging through server logs - but you need access to the file structure of the server for this (usually via FTP). If you do not have access to the server, you will have to display error messages on the website, which is usually rather undesirable. In the case of PHP errors, the browser may also only display an empty site - PHP errors are therefore all the more serious for your website. If you use WordPress, WP Debug can help you here.
Here is another difference between JavaScript and PHP - when your JavaScript code contains an error, only a single element may not work. When the PHP script contains errors, you will normally see nothing but a white screen (unless you have enabled error messages on the website).
By the way, it can lead to errors in PHP files if the tag ?> is left at the very end. The tag ?> should be omitted if the file consists only of PHP code.
Conclusion
PHP is a somewhat more complicated language. But it's worth learning - at least if you wish to do more than just use ready-made WordPress themes and plugins. Or simply if you have reached your limits with JavaScript, HTML and CSS. If you want to change your WordPress theme or write your own plugins, you can't get around PHP. You also need PHP for all database matters - and even SQL, at least if you want to stick to the standard. If you like to learn PHP, there is a tutorial available.
Your questions about PHP
Do you have any questions for Patrick? Feel free to use the comment function. You want to be informed about new posts on the topic of WordPress and web design? Then follow us on Twitter, Facebook, LinkedIn or via our newsletter.